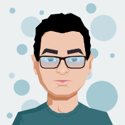
Published 2022-01-03 23:32:24
Assert Statement
Assert Statement
The assert statement in Python is primarily used to test that your code returns the expected results.
This is the official syntax for the assert statement (you can read it here):
assert_stmt ::= "assert" expression ["," expression]
The assert statements are usually meant for the developers to easily find the errors and to debug the code, but it doesn't provide anything useful to the end-users. As long as your code is error free, you don't need to worry, in contrary it can stop your application from running.
Let's assume this very simple sum calculator function that takes any number of arguments and returns the sum of all numbers.
Also , we have added an assert statement to check that results will never be lower than 10 or higher than 20.
def sum_calc(*args):
assert 10 <= sum(args) < 20
return sum(args)
result = sum_calc(1, 2 ,3 ,10)
print(result)
Output:
16
If we now add another argument of 5, what will the result be?
def sum_calc(*args):
assert 10 <= sum(args) < 20
return sum(args)
result = sum_calc(1, 2 ,3 ,10, 5)
print(result)
Since the assert statement doesn't allow something to be higher than 20, the following error would be thrown:
Traceback (most recent call last):
File "assert_1.py", line 18, in <module>
result = sum_calc(1, 2 ,3 ,10, 5)
File "assert_1.py", line 15, in sum_calc
assert 10 <= sum(args) < 20
AssertionError
The same goes if the sum is lower than 10.
Assume we have the following list of numbers
result = sum_calc(1, 2 ,3 )
We'd get the exact same error message
Traceback (most recent call last):
File "assert_1.py", line 18, in <module>
result = sum_calc(1, 2 ,3 ,10, 5)
File "assert_1.py", line 15, in sum_calc
assert 10 <= sum(args) < 20
AssertionError
The following form is equivalent to the Assert statements
def sum_calc(*args):
# assert 10 <= sum(args) < 20, "Error - Beyond the boundaries"
if __debug__:
if not 10 >= sum(args) or sum(args) > 20:
raise AssertionError("Error - Beyond the boundaries")
return sum(args)
result = sum_calc(1, 2 ,3, 20)
__debug__ is enabled by default , you can check it by typing the dunder method in the Python interpreter:
__debug__
True
It is possible to disable the Assert by running Python with the command line option -o
python -O
Now you can check the state of __debug__
__debug__
False
Custom error message
You can add an additional argument with your custom message
def sum_calc(*args):
assert 10 <= sum(args) < 20, "Error - Beyond the boundaries"
return sum(args)
If we re-run our faulty app, the output would be like this:
AssertionError: Error - Beyond the boundaries
Summary
- Asserts are good for developers to find the errors in their code.
- The recommendation is not to use Asserts for data validations, it's better to actually have other kinds of checks, like with old good if..else statements.
- I'd also argue the asserts are statements are not good for production code in many cases.
- pytest is a great framework for creating tests and you can use assertions to validate data and other things. We will cover it in an upcoming course, stay tuned.