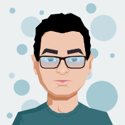
Tutorial: Storing and Using a Custom Environment Variable in GitHub Secrets with AWS CDK
This guide will walk you through the process of securely storing a custom environment variable in GitHub Secrets and accessing it within an AWS Cloud Development Kit (CDK) deployment.
For this example, we will use the variable name CUSTOM_OIDC_CLIENT_ID
.
Pre-requisites
To ensure that you can successfully follow this tutorial, you should meet the following requirements:
- Existing AWS CDK Stack:
You should have an existing AWS CDK stack that you are currently managing or developing. This stack is the basis for integrating the secure handling of sensitive environment variables. - Familiarity with AWS CDK:
A working knowledge of AWS Cloud Development Kit (CDK) is crucial. You should be comfortable with the basic concepts of CDK, such as stacks, constructs, and the CDK CLI. - GitHub Account and Repository:
A GitHub account with a repository where your AWS CDK code is stored. This tutorial uses GitHub Actions and Secrets to securely manage and deploy your CDK application. - Basic Understanding of GitHub Actions:
Understanding how to configure and use GitHub Actions is essential since we will modify the CI/CD workflow to include secrets.
Goal of the Tutorial
By the end of this tutorial, you will be able to:
- Store sensitive data securely using GitHub Secrets.
- Access and use these secrets within your AWS CDK stack to deploy resources securely.
- Understand best practices for managing sensitive configuration data outside of your codebase to enhance the security posture of your infrastructure deployments.
Step 1: Setting up GitHub Secrets
To begin, you will store the custom OIDC client ID in GitHub Secrets to secure the sensitive information.
- Navigate to Your GitHub Repository: Go to the GitHub repository where your AWS CDK code is housed.
- Access the Settings Tab: Click on the 'Settings' tab on the repository's homepage.
- Select Secrets: Choose 'Secrets' from the left sidebar, then select 'Actions'.
Add a New Secret: Click on 'New repository secret'. - Name Your Secret: Enter
CUSTOM_OIDC_CLIENT_ID
as the name for your secret. - Set the Secret Value: Type in the value for your custom OIDC client ID.
Save the Secret: Click 'Add secret' to store it securely.
Step 2: Modifying GitHub Actions Workflow
Update your GitHub Actions workflow to use the newly created secret during the deployment process.
Here is an example of a workflow file:
jobs:
deploy_job:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
- name: Setup Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Deploy CDK Stack
env:
CUSTOM_OIDC_CLIENT_ID: ${{ secrets.CUSTOM_OIDC_CLIENT_ID }}
run: npm run deploy-custom-stack ${{ inputs.stack }}-DEV
In this setup, the CUSTOM_OIDC_CLIENT_ID
environment variable is populated with the secret value.
Step 3: Accessing the Secret in AWS CDK
Modify your AWS CDK application to use the custom environment variable during deployment.
Edit the CDK deployment command in package.json:
Ensure the CDK deployment command includes the new environment variable.
- Edit the CDK deployment command in
package.json
and ensure the CDK deployment command includes the new environment variable:
"scripts": { "deploy-custom-stack": "cdk deploy --require-approval never --context CUSTOM_OIDC_CLIENT_ID=$CUSTOM_OIDC_CLIENT_ID" }
- Update the CDK Stack Code (
my-custom-cdk-stack.ts
): Utilize the context variable to pass the client ID to your CDK stack:
import { Stack, Construct } from '@aws-cdk/core'; export class MyCustomCDKStack extends Stack { constructor(scope: Construct, id: string, props) { super(scope, id, props); // Retrieve the custom OIDC client ID from context const oidcClientId = this.node.tryGetContext('CUSTOM_OIDC_CLIENT_ID'); // Assume props.OIDCInfo is available from passed props new MyOIDCResource(this, 'OIDCResource', { oidc: { ...otherstuff, clientId: oidcClientId, } }); } }
You probably have some other parameters that we refer to as otherstuff
By integrating the custom environment variable in this manner, you securely pass sensitive information into your deployment without exposing it in your codebase.
Conclusion
This method ensures your sensitive data, like an OIDC client ID, is handled securely using GitHub Secrets and AWS CDK, thereby protecting your infrastructure from potential security vulnerabilities. This approach is essential for maintaining robust security practices in cloud application deployments.
Please let me know in the comment if you want a more detailed and complete tutorial about this topic.