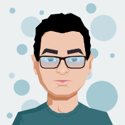
Python - You need to know Map() Function!
thanks to freepik for wonderful images
#pythonlearning #python #pythonprogramming #programming
Introduction
The map()
function is a tool in Python that enables you to efficiently apply a function to each element in an iterable object and return a new, transformed iterable. With map()
, you can take any iterable - such as a list, tuple, or set - and apply a custom function, class, or method to each element, creating a new iterable that contains the results of these transformations. To use map()
, you simply provide two arguments: the function that you want to apply, and the iterable that you want to transform. This powerful function can simplify your code and make it more readable and maintainable, making it a valuable tool in any Python programmer's toolkit.
In this article, we will dive into the powerful map()
function in Python and learn about its functionality, uses, and how to combine it with other functions such as filter()
and reduce()
. We will also take a closer look at how to use lambda functions with map()
to solve different use cases in a more efficient and elegant way.
By the end of this article, you will have a fundamental understanding of the map()
function, its capabilities, and how to use it to make your own code more concise and efficient. So let's get started and see how we can harness the power of map() in Python.
Content
The following is the content of the article:
- Prerequsites
- Analogy of map() function
- What is map() function in programming
- Examples
- Manipulate Strings with map() function
- Combine map() function with some other functions
- Summary
Prerequsites
Some basic Python knowledge is good to have.
Analogy
Think of the map()
function as a machine that takes two inputs: a function and an iterable object. The function acts as the recipe or blueprint, and the iterable object is the ingredients. The machine applies the recipe to each ingredient, and produces a new, transformed iterable object as the output, similar to how a bakery would use a recipe to make multiple cakes from the same ingredients.
What is a map() Function In Programming?
The map()
function takes two arguments: a function and an iterable object. The function is applied to each element in the iterable, and the results of each function application are collected and returned as a new iterable object, usually a map object. The type of the returned iterable object depends on the type of the iterable passed as the second argument.
While you can achieve similar results using a for loop with an iterable such as a list or tuple, there are several advantages to using the map()
function instead:
-
Concise code: The
map()
function can accomplish the same task in a single line of code, making your code more readable and concise -
Reusability: The same function can be used to process different iterables, making it a more flexible option
-
Multiple iterables: The
map()
function can accept multiple iterables, allowing you to process multiple sequences at once. However, the number of iterables must be accepted by the function being passed tomap()
-
Lambda compatibility: The
map()
function can be used with disposable lambda functions, providing another way to simplify your code
Examples
Example - add sum
This is our most simplest example that we will start with.
In the following example, we have a list called numbers
that contains integer values. The add
function takes an integer argument x
and returns x + 2
.
Using the map
function, we apply the add
function to every item in the numbers
list, resulting in a new list of the modified values.
This new list is assigned to the result
variable.
So, for example, the first item in the numbers
list, 1, is passed to the add
function as an argument, and the function returns 3, which becomes the first item in the result
list. This process is repeated for each item in the numbers
list:
def add(x):
return x + 2
numbers = [1, 2, 3, 4, 5]
result = list(map(add, numbers))
print(result)
Output:
[3, 4, 5, 6, 7]
Use Case - Salaries
A company wanted to give its employees a raise. The company had a list of salaries for each employee and wanted to increase each salary by 2%. The HR team asked us to write a code to calculate the new salaries for each employee.
Try to solve it using the map()
function.
Solution to Salaries Use Case
Let's check one simple possible solution using map()
function
In the following example, we've written a salary_increase
function that takes an employee's salary as an input and returns the increased salary. The function multiplies the salary by 0.02 to calculate the 2% increase and then adds the original salary to the result.
We're using the map
function to apply the salary_increase
function to each salary in the salaries
list. The map
function returns a map object, so we have to use list
function to convert the map object to a list and store it in a new list called new_salaries
'''
devoriales.com, 2023
Path: map_function/salary.py
description: map function
'''
# Python program to increase salaries with map() function and return new salaries
# Return increased salaries
def salary_increase(n):
return n * 0.02 + n
salaries = [60000, 50000, 8000, 3000] # list of salaries
new_salaries = list(map(salary_increase, salaries)) # map() function returns a map object, so we need to convert it to a list
print(new_salaries) # print new salaries
Output:
[61200.0, 51000.0, 8160.0, 3060.0]
Let's now use the Lamda function to see how that works, we will just comment out part of the previous code:
'''
devoriales.com, 2023
Path: map_function/salary.py
description: map function
'''
# Python program to increase salaries with map() function and return new salaries
# Return increased salaries
def salary_increase(n):
return n * 0.02 + n
salaries = [60000, 50000, 8000, 3000] # list of salaries
# map() function returns a map object, so we need to convert it to a list
# new_salaries = list(map(salary_increase, salaries))
# print(new_salaries) # print new salaries
# example with lambda function
new_salaries = map(lambda n: n * 0.02 + n, salaries)
print(list(new_salaries)) # print new salaries
Basically the functionallity of the code is the same as in the previous example, but this time we're using lambda function.
The lambda
function takes one argument, n
, and returns the result of n * 0.02 + n
, which is a simple mathematical expression that adds 2% to each element of the salaries
list.
The map
function returns a map object, which can be converted to a list by passing it to the list
function. The result is a new list, new_salaries
, which contains the modified salary values.
So in short, the code you provided creates a new list of salaries by applying a 2% raise to each salary in the original salaries
list.
Output (same result as before):
[61200.0, 51000.0, 8160.0, 3060.0]
Manipulate strings with map()
The map() function can be useful for working with string objects in Python. Before we dive into some examples, it's important to understand the methods available in the str class.
Python has a built-in class called str, and with its string methods, we can perform many string manipulations. Here are some of the most common methods:
str.strip([chars])
: Returns a copy of the string with leading and trailing whitespace removed.str.capitalize()
: Returns a capitalized version of the string.str.lower()
: Returns a copy of the string converted to lowercase.str.upper()
: Returns a copy of the string converted to uppercase.str.title()
: Returns a version of the string where each word is title cased.str.swapcase()
: Converts uppercase characters to lowercase and lowercase characters to uppercase.
It's important to note that these methods return a copy of the string. This is because string objects are immutable once created, similar to tuples. When a string method is called, Python creates a new string object as a result.
Let's examine how we can use the map()
function to manipulate string objects.
Consider the following example where we create a list of car names with some unwanted whitespaces:
# Here we create a list of cars.
cars = ['Porsche ', 'Bmw', ' Audi', ' Volvo ', 'Mazda ', 'Peugeot']
We can now use the map()
function to process this list by passing it to the str.strip()
method. It's important to convert the map object to a list object, as the result of the map object won't be visible otherwise. We pass the list of our cars to the str.strip()
method and don't provide any arguments to the strip()
method since the default behavior is to remove white spaces:
cleaned_list = list(map(str.strip, cars))
Let's print out the cleaned list:
print(cleaned_list)
The whitespaces in the output have been stripped away:
['Porsche', 'Bmw', 'Audi', 'Volvo', 'Mazda', 'Peugeot']
Example - The Caesar Cipher
The Caesar Cipher is one of the oldest encryption techniques that we know about.
A cite from Wikipedia: "The Caesar cipher is named for Julius Caesar, who used an alphabet where decrypting would shift three letters to the left."
Plain | A | B | C | D | E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U | V | W | X | Y | Z |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Cipher | X | Y | Z | A | B | C | D | E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U | V | W |
Let's look at an example of using map()
to create a small encryption application. We will use the string module to shift each letter in a given string by three places.
The map() function will allow us to apply this shift to each letter in the string easily and efficiently.
We are going to use the string
module in our example to manipulate strings. Specifically, we are importing the ascii_lowercase
as alphabet
which provides us with the 26 lowercase ASCII characters and we can use this to encode our messages:
from string import ascii_lowercase as alphabet
print(alphabet)
Output:
abcdefghijklmnopqrstuvwxyz
Now let's add the following function to our code.
This following code imports the ascii_lowercase
constant from the string
module and uses it as alphabet
for the encryption.
The encrypt
function takes in a letter
and applies a Caesar cipher to it with a shift of 3. The function first converts the letter
to lowercase and checks if it exists in alphabet
. If it does not, it returns the original letter. If it does, it finds the index of the letter in alphabet
, shifts it by 3, and takes the modulo of the length of alphabet
to handle the wrap around. Finally, it returns the letter at the new index in alphabet
.
The code then applies the encrypt
function to each letter in the my_code
string using the map
function and converts the resulting map object to a list using the list
function. The list is then joined into a single string using the join
method.
'''
devoriales.com, 2023
Path: map_function/caesar_encryption.py
description: caesar encryption with map function
'''
from string import ascii_lowercase as alphabet
def encrypt(letter):
shift = 3
letter = letter.lower()
if letter not in alphabet: # alphabet = 'abcdefghijklmnopqrstuvwxyz'
return letter
# check the index of the letter in the alphabet and add the shift value. If the new index is greater than the length of the alphabet, start from the beginning
new_letter = (alphabet.index(letter) + shift) % (len(alphabet))
return alphabet[new_letter] # return the letter in the alphabet with the new index
my_code = 'My Secret Message'
encrypt = list(map(encrypt, my_code))
print("".join(encrypt))
# print(encrypt)
❗Please Note! The operator %
is the modulus operator in Python. It returns the remainder of the division of one number by another. In the line new_letter = (alphabet.index(letter) + shift) %(len(alphabet))
, %(len(alphabet))
is used to wrap the result of the calculation (alphabet.index(letter) + shift)
around the length of the alphabet
string. This allows for the result to be within the range of indices of alphabet
, even if the sum of alphabet.index(letter)
and shift
is greater than len(alphabet)
.
And the output we get is:
pb vhfuhw phvvdjh
Now if you compare it with the table, you will see that our encryption works just fine
Plain | A | B | C | D | E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U | V | W | X | Y | Z |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Cipher | X | Y | Z | A | B | C | D | E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U | V | W |
Combine map() function with some other functions
filter()
function used together with map()
function
Introduction to filter()
function
The filter()
function in Python is used to filter out elements from a given iterable (e.g. list, tuple, etc.) that do not meet a specified condition. It takes two arguments: a function and an iterable. The function is a test that returns either True
or False
, and the iterable is the input data that we want to filter.
The filter()
function returns an iterator that provides elements from the iterable for which the test function returns True
. The returned object is an iterable filter object, which can be converted to other Python objects (e.g. list) using the list()
or tuple()
functions.
Just like map()
, the filter()
function takes two positional arguments:
filter(function, iterable)
The function argument must return a Boolean value of True or False.
Using the filter()
function in combination with the map() function is an effective way to filter results based on a True
or False
condition.
In this example below, the is_even()
function takes an element of the numbers
list as input and returns True
if the number is even, and False
if it's not.
The filter()
function is then used to filter the numbers
list using this test function, and the result is a filter object that only contains even numbers.
This filter object is then converted to a list using the list()
function:
def is_even(num):
return num % 2 == 0
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = filter(is_even, numbers)
print(list(even_numbers))
# Output: [2, 4, 6, 8, 10]
Example - Check bath weather
Your family members were interested in finding out which days of the year had temperatures higher than 68°F in a specific location, which they referred to as "Bath weather." They asked you to come up with a solution and, as a result, gathered weather data for different months of the year, including the date and temperature for each day.
We need to write a code that calculates the days with temperatures above a specified value (68°F) and converts those temperatures to Celsius.
You will write a check_weather
function that needs to accept a tuple of month, day, and temperature in Fahrenheit, and it should return True
if the temperature is greater than the specified value. Next, you will write a convert
function that accepts a tuple, unpacks it, converts the temperature to Celsius, and returns a tuple with the updated temperature. The weather_data
list should be filtered using the check_weather
function, and the temperatures in the filtered data should be converted to Celsius using the convert
function. Finally, the resulting converted data should be printed, displaying the month, day, and temperature in Celsius.
Let's solve this problem:
We have a list of tuple objects, each representing the day, month, and temperature in°F:
daily_temp = [('July', '1', 65), ('July', '2', 72), ('July', '3', 66), ('July', '4', 75), ('July', '5', 79)]
The formula for converting Fahrenheit to Celsius is
So let's add a function that converts °F to °C
def check_weather(mdt): # we accept tuple here
bath_weather = 68 # setting or accepted bath_weather temperature
m, d, t = mdt # now we unpack each item in the iterable
return t > bath_weather # and let's return just if temperature that day is above our accepted bath temperature
Now let's create one more function to convert the temperature to Celsius, so we don't freeze to death:
def convert(mdt): # we accept tuple here, like we did in check_weather()
m, d, t = mdt # Let's unpack the values in the tuple
t = tuple(map(lambda x: round((t-32) * 0.5556, 2), mdt)) # converting the temperature (t) with lambda function
t = t[2] # Here we assign the index of 2 from t variable. Index 2 is the third position
mdt = m, d, t # assigning the values back, now with t that has Celcius measure
return mdt
Let's create a variable called hot_weather
that contains the tuples and applies the convert()
function. As a second argument, we will insert the filter()
function, which will only return those tuples that have a temperature higher than the temperature specified in the hot_weather
variable, as determined by the check_weather()
function:
hot_weather = map(convert, filter(check_weather, daily_temp))
Now let's print out our hot_weather variable as a list. Again, please remember, hot_weather is iterable, so we have to assign an iterable type:
print(list(hot_weather))
Output:
[('July', '2', 22.22), ('July', '4', 23.89), ('July', '5', 26.11)]
Complete Code:
'''
devoriales.com, 2023
Path: map_function/map_and_filter.py
description: map and filter functions
'''
def check_weather(mdt): # we accept tuple here
bath_weather = 68 # setting or accepted bath_weather temperature
m, d, t = mdt # now we unpack each item in the iterable
# and let's return just if temperature that day is above our accepted bath temperature
return t > bath_weather
def convert(mdt): # we accept tuple here, like we did in check_weather()
m, d, t = mdt # Let's unpack the values in the tuple
# converting the temperature (t) with lambda function
t = tuple(map(lambda x: round((t-32) * 0.5556, 2), mdt))
# Here we assign the index of 2 from t variable. Index 2 is the third position
t = t[2]
mdt = m, d, t # assigning the values back, now with t that has Celcius measure
return mdt
weather_data = [
('Jan', '1', 32),
('June', '25', 75),
('July', '4', 100),
('Aug', '15', 92),
('Sept', '22', 88),
('Oct', '31', 72),
]
filtered_data = list(filter(check_weather, weather_data))
# print('filtered_data:', filtered_data)
# Let's convert the temperature to Celcius
converted_data = list(map(convert, filtered_data))
print('Bath weather days:')
for m, d, t in converted_data:
print(f'{m} {d}: {t}°C')
Output (so even normal people, not coder, can read 🙂):
Bath weather days:
June 25: 23.89°C
July 4: 37.78°C
Aug 15: 33.34°C
Sept 22: 31.11°C
Oct 31: 22.22°C
reduce()
function used together with map()
function
Introduction to reduce()
function
The reduce()
function is a built-in function in Python that belongs to the functools
module. It is used to apply a particular function to a sequence of elements, cumulatively reducing the sequence to a single value. The reduce
function operates by applying the given function to the first two elements of the sequence, then using the result as the first argument to apply the function to the next element, and so on.
In other words, the reduce()
function takes a function and a sequence as input, and then applies the function to the elements of the sequence in a cumulative manner. It starts by combining the first two elements of the sequence, then combines the result with the third element, and so on, until all elements have been combined into a single value.
This function can be used to perform tasks such as finding the product of all elements in a list, computing the greatest common divisor of a list of numbers, or calculating the sum of squares of all elements in a list. The reduce()
function is a powerful tool for performing complex operations on sequences.
In the following diagram example, the reduce()
function is applied to a list of numbers [1, 2, 3, 4]
.
The reduce()
function starts by taking the first two elements in the list [1, 2]
and applying a function to them, then it takes the result 3
and applies a function again with the next number 3
, and so on until it has processed all the numbers in the list. In this case, the final result of the reduce()
function is 10
which is the sum of all the numbers in the list.
The result is 10
A Python code could look like the following:
numbers = [1, 2, 3, 4]
sum_of_numbers = reduce(lambda x, y: x + y, numbers) # 1 + 2 + 3 + 4 = 10
print(sum_of_numbers)
Output:
10
Example - calculate total square meters of all the rooms in the building
Use Case:
When the construction of a new building was underway, the builder had a problem to solve. He needed to calculate the total square meters of all the rooms in the building. The builder had a list of measurements for each room that included the name of the room, the length, and the width.
He asked us to help him with the calculation using some sorts of computer program.
Based on the input we got from the builder, we started by creating a list of tuples that has all required information for all rooms:
measurements = [
('room1', 10, 10),
('room2', 10, 10),
('room3', 10, 10),
('room4', 10, 10),
('room5', 10, 10),
]
We then created a function that would calculate the square meters of each room. The function would take in one measurement, unpack the length and the width, multiply them, and return the square meters. We call this function "calculate_square_meters".
# we will use map function to calculate the square meters of each room
def calculate_square_meters(measurement):
name, length, width = measurement # unpack the tuple
square_meters = length * width # calculate the square meters
return square_meters # return the square meters
Next, we used the map()
function to apply the calculate_square_meters()
function to each measurement in the list. This would give us a list of square meters for each room:
# with reduce function we can sum all the square meters. we will use lambda function
total_square_meters = reduce(lambda x, y: x + y, map(calculate_square_meters, measurements))
The map()
function is applied to the list of room measurements (the measurements
variable) and the calculate_square_meters
function. The calculate_square_meters
function takes one measurement tuple and calculates the square meters of that room. The map function applies the calculate_square_meters
function to each measurement tuple in the measurements
list, returning a list of square meters of each room.
The reduce()
function is then used to sum up all the square meters in the list. The reduce function takes two arguments: a lambda function lambda x, y: x + y
and the output of the map function. The lambda function adds the current item x
with the next item y
in the list, and the reduce function applies this lambda function cumulatively to all items in the list, reducing the list to a single value, which is the sum of all the square meters.
The final result of the code is stored in the total_square_meters
variable.
Final code:
'''
devoriales.com, 2023
Path: map_function/map_reduce.py
description: map and reduce functions
'''
from functools import reduce
# sum of all room square meters based on measurements
measurements = [
('room1', 10, 10),
('room2', 10, 10),
('room3', 10, 10),
('room4', 10, 10),
('room5', 10, 10),
]
# we will use map function to calculate the square meters of each room
def calculate_square_meters(measurement):
name, length, width = measurement # unpack the tuple
square_meters = length * width # calculate the square meters
return square_meters # return the square meters
# with reduce function we can sum all the square meters. we will use lambda function
total_square_meters = reduce(lambda x, y: x + y, map(calculate_square_meters, measurements))
Output:
total_square_meters: 500
Summary
In this lesson on the map() function, we covered the following topics:
- Understanding what the
map()
function is and what it aims to solve - How to pass iterators to a function using the
map()
function - Using lambda functions with
map()
functions - Combining the
map()
andfilter()
functions - Combining the
map()
andreduce()
functions - Solving various use cases with the
map()
function
By the end of this lesson, you should have a clear understanding of how you can use the map()
function in your own code.
About the Author
Aleksandro Matejic, a Cloud Architect, began working in the IT industry over 21 years ago as a technical specialist, right after his studies. Since then, he has worked in various companies and industries in various system engineer and IT architect roles. He currently works on designing Cloud solutions, Kubernetes, and other DevOps technologies.
In his spare time, Aleksandro works on different development projects such as developing devoriales.com, a blog and learning platform launching in 2022/2023. In addition, he likes to read and write technical articles about software development and DevOps methods and tools. You can contact Aleksandro by visiting his LinkedIn Profile.