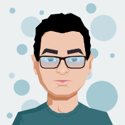
Python - Data Structure Types - Sets
Python - Data Structure Types - Sets
Content
Introduction
What methods can we use with Python sets?
Method - add()
Method - remove()
Method - difference()
Method - intersection()
Method - symmetric_difference()
Method - pop()
Method - union()
Method - update()
Conclusion
Introduction
Sets are another data structure type in Python. I find that people generally underestimate the power of sets, and there are some really cool cases for this data structure type.
Compared to lists, sets are defined with curly braces, but you can also use the set function. Let's see some examples:
car_manufacturers = {'Porsche', 'Bmw', 'Audi', 'Volvo', 'Audi'}
and then let's print it out:
>>> print(car_manufacturers)
{'Volvo', 'Audi', 'Porsche', 'Bmw'}
A question. What do you notice in the output?
The following defines a set:
- A set does not allow duplicates. We added 'Audi' two times, but it was only printed out once.
- A set does not sort the items, the order is never guaranteed.
- You can update a set, but the items in a set are immutable.
What methods can we use with Python sets?
Sets provide some more cool features. We won't cover them all, but let's look at some of them.
The easiest way to print out the methods supported by the set is with the following command:
>>> dir(set)
['__and__', '__class__', '__contains__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__iand__', '__init__', '__init_subclass__', '__ior__', '__isub__', '__iter__', '__ixor__', '__le__', '__len__', '__lt__', '__ne__', '__new__', '__or__', '__rand__', '__reduce__', '__reduce_ex__', '__repr__', '__ror__', '__rsub__', '__rxor__', '__setattr__', '__sizeof__', '__str__', '__sub__', '__subclasshook__', '__xor__', 'add', 'clear', 'copy', 'difference', 'difference_update', 'discard', 'intersection', 'intersection_update', 'isdisjoint', 'issubset', 'issuperset', 'pop', 'remove', 'symmetric_difference', 'symmetric_difference_update', 'union', 'update']
We're going to cover a number of those methods
Method - add()
With add() function, we can add new elements to an existing set. In this example, we're going to add Peugeot to our set.
>>> car_manufacturers.add('Peugeot')
>>> print(car_manufacturers)
{'Porsche', 'Bmw', 'Audi', 'Volvo', 'Peugeot'}
Method - remove()
>>> car_manufacturers.remove('Peugeot')
>>> print(car_manufacturers)
{'Volvo', 'Bmw', 'Audi', 'Porsche'}
Method - difference()
Now let's add another set:
car_manufacturers_two = {'Fiat', 'Alfa Romeo', 'Opel', 'Mazda', 'Toyota', 'Audi'}
so with difference()
we can now compare our two sets:
>>> car_manufacturers.difference(car_manufacturers_two)
{'Volvo', 'Porsche', 'Bmw'}
Method - intersection()
With intersection()
method we can return the item that exists in both sets:
>>> car_manufacturers.intersection(car_manufacturers_two)
{'Audi'}
Method - symmetric_difference()
With this function, we can add the second set to the first one and get a symmetric difference between both sets:
>>> car_manufacturers.symmetric_difference(car_manufacturers_two)
{'Volvo', 'Opel', 'Bmw', 'Alfa Romeo', 'Mazda', 'Fiat', 'Toyota', 'Porsche'}
Method - pop()
With pop() we remove an arbitrary element from the set. This means that a random element gets removed.
>>> car_manufacturers.pop()
'Volvo'
Method - union()
Explanation: Create a new set by merging two existing sets
>>> car_manufacturers_three = car_manufacturers.union(car_manufacturers_two)
>>> print(car_manufacturers_three)
{'Alfa Romeo', 'Mazda', 'Opel', 'Fiat', 'Toyota', 'Bmw', 'Audi', 'Porsche'}
Method - update()
Explanation: Update one set with another set.
>>> car_manufacturers.update(car_manufacturers_two)
>>> print(car_manufacturers)
{'Alfa Romeo', 'Mazda', 'Opel', 'Fiat', 'Toyota', 'Bmw', 'Audi', 'Porsche'}
Conclusion
Essentially, sets are really nice if we want to avoid duplicates, which is not the case with the lists. But as we saw in the provided examples, sets also provide some very useful methods that we can use in our code. Hopefully, now, you have some basic understanding of what the set is and how to use them in your code. We'll cover sets even further in our Python Fundamental Course