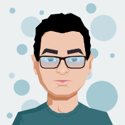
The difference between var, let and const variable declarations
The difference between var, let and const variable declarations
Introduction
This short article describes the differences between var
, let
and const
keywords when used to declare variables in JavaScript.
It is essential to understand the difference since the use case and behaviors are different.
❗var
was the only variable declaration before ECMAScript 6. This has changed since it was really hard to keep track of since it could be re-declared later in the code inside a block and caused challenges when trying to find where the declaration and value assignment occurred. To declare a variable with let
keyword has become a much better practice.
Declaration vs Assignment
In this article, we use declaration and assignment terminologies.
What we mean is:
declaration: The declaration gives a name to the variable. Unique when referring to.
assignment: Assigning an initial value, which can be any data type, to the variable.
Differences between let, const and var
The following section is explaining var
, let
and const
variable declaration keywords.
var declaration
- can be declared after the assignment (yeah can be confusing)
- Variable value can be reassigned
- variable can be re-declared
- accessible outside a block (yet another complexity)
- not recommended to be used anymore, use
let
andconst
instead.
In the following example, we will declare the varVariable
inside a block and try to access it from outside a block:
if (1 === 1) {
var varVariable = 'this is varVariable' // can be re-declared, can be declared after assigning
}
console.log(varVariable) // accessable outside the block
Output:
this is varVariable
Worked, it's accessible from outside the block.
In the following example, we will try to change the value of the variable:
if (1 === 1) {
var varVariable = 'this is varVariable' // can be re-declared, can be declared after assigning
varVariable = 're-assign a new value'
}
console.log(varVariable) // accessible outside the block
Output:
re-assign a new value
It worked. And now we will try to re-declare the variable:
if (1 === 1) {
var varVariable = 'this is varVariable' // can be re-declared, can be declared after assigning
varVariable = 're-assign a new value' //can assign a new value
}
var varVariable = 'varVariable re-declared' // can be re-declared, can be declared after assigning
console.log(varVariable) // accessible outside the block
Output:
varVariable re-declared
let declaration
- variable declared inside a block is available only within a block.
- You can assign a new value
- cannot be re-declared after the assignment
In the first example, we will try to access the variable outside a block:
if (1 === 1) {
let letVariable = 'this is letVariable' // only within the block, can be re-declared. Has to be declared before been used
}
console.log(letVariable)
Output:
Uncaught ReferenceError: letVariable is not defined
If we move console.log()
inside a block, it will work:
// console.log inside the block
if (1 === 1) {
let letVariable = 'this is letVariable' // only within the block, cannot be re-declared. Has to be declared before used
console.log(letVariable)
}
Output:
this is letVariable
We were able to access it from the code block only.
Now let's check if we can re-assign another value to the letVariable
variable:
if (1 === 1) {
let letVariable = 'this is letVariable' // only within the block, can be re-declared. Has to be declared before used
letVariable = 'this is letVariable but different' // assigning another string
console.log(letVariable)
}
Output:
this is letVariable but different
We were able to assign a new value the letVariable
And now, let's try to re-declare the variable:
if (1 === 1) {
let letVariable = 'this is letVariable' // accessible only within the block
let letVariable = 'this is letVariable but different' // re-declaring
console.log(letVariable)
}
Output:
Uncaught SyntaxError: Identifier 'letVariable' has already been declared
const declaration
- variable declared inside a block is available only within a block.
- It cannot be re-declared
- variable cannot change its value after the initial assignment.
Trying to access a var outside a block:
if (1 === 1) {
const constVariable = 'this is constVariable' // accessible within the block only, cannot be re-declared
}
console.log(constVariable)
Output (Error):
Uncaught ReferenceError: constVariable is not defined
Now let's output it inside a block:
if (1 === 1) {
const constVariable = 'this is constVariable' // accessible within the block only, cannot be re-declared
console.log(constVariable)
}
Output:
this is constVariable
And finally, we'll try to change the string assigned to the variable:
if (1 === 1) {
const constVariable = 'this is constVariable' // accessible within the block only, cannot be re-declared
constVariable = 'this is constVariable but different'
console.log(constVariable)
}
Output (Error):
Uncaught TypeError: Assignment to constant variable.
The major difference between let
and const
is, the latter cannot change its value. Great if you want to make sure that the value is consistent across the code.
Summary
In this article, we learned the differences between var
, let
, and const keywords when declaring a variable.
We learned the following
- var:
- can be re-declared
- can be declared after the assignment (yeah can be confusing)
- can change the value
- accessible outside a block
- not recommended to be used anymore
- let:
- variables only available inside a block
- Cannot be re-declared
- you can assign another value to the variable
-
- const:
- declared variables only available inside a block
- variables cannot be re-declared
- you cannot assign another value to the variable
- good safeguard
A recommendation is to use const
keyword if you don't want the variable to change its value.
If you want to allow a variable to get changed, use let
keyword. And avoid var
keyword, there is no need to use it anymore. It's basically extremely hard to troubleshoot since a declared variable inside a block is accessible even outside a block. And the value can change too. And also, you can re-declare the variable.
About the Author
Aleksandro Matejic, Cloud Architect, began working in IT Industry over 20y ago as a technical consultant at Lindahl Rothoff in southern Sweden. Since then, he has worked in various companies and industries like Atea, Baxter, and IKEA having various architect roles. In his spare time, Aleksandro is developing and running devoriales.com, a blog and learning platform launched in 2022. In addition, he likes to read and write technical articles about software development and DevOps methods and tools. You can contact Aleksandro by paying a visit to his LinkedIn Profile.