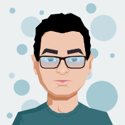
Understanding Python Assert: When to Use It and When to Avoid It
The assert
statement in Python is a debugging tool that tests whether a condition in your code is True. If the condition is False, Python raises an AssertionError
exception and stops execution. While useful for development and testing, assert statements require careful consideration regarding their proper use cases.
The Basic Syntax
The official syntax for the assert statement is:
assert_stmt ::= "assert" expression ["," expression]
This means you can write either:
assert condition
- Raises AssertionError if the condition is Falseassert condition, "error message"
- Raises AssertionError with a custom message
Simple Example: Sum Calculator
Let's examine a practical example with a sum calculator function that validates its output:
def sum_calc(*args):
assert 10 <= sum(args) < 20
return sum(args)
result = sum_calc(1, 2, 3, 10)
print(result) # Output: 16
The assert statement checks that the sum falls within the range of 10 to 20 (inclusive of 10, exclusive of 20).
When the Assert Fails
If we exceed the boundaries:
result = sum_calc(1, 2, 3, 10, 5) # Sum = 21
Python throws:
Traceback (most recent call last):
File "assert_1.py", line 18, in <module>
result = sum_calc(1, 2, 3, 10, 5)
File "assert_1.py", line 15, in sum_calc
assert 10 <= sum(args) < 20
AssertionError
Similarly, if the sum is too low:
result = sum_calc(1, 2, 3) # Sum = 6
You'll get the same error message.
What Happens Behind the Scenes
Assert statements are equivalent to this conditional code:
def sum_calc(*args):
if __debug__:
if not (10 <= sum(args) < 20):
raise AssertionError("Error - Beyond the boundaries")
return sum(args)
The __debug__
flag is True by default. You can check its value in any Python interpreter:
print(__debug__) # Output: True
Disabling Assertions
You can disable assertions entirely by running Python with the -O
(optimize) flag:
python -O your_script.py
After this, checking __debug__
returns:
print(__debug__) # Output: False
Custom Error Messages
Adding meaningful error messages helps with debugging:
print(__debug__) # Output: False
When the assertion fails, you'll see:
AssertionError: Error - Beyond the boundaries
Best Practices and Recommendations
✅ When to Use Assert
- During development to catch bugs early
- For testing internal invariants that should never be False
- When debugging complex logic
- In unit tests (though testing frameworks like
pytest
are preferred)
❌ When to Avoid Assert
- For user input validation
- For production data validation
- For handling business logic errors
- For conditions that might naturally occur during runtime
Instead of assertions, use explicit error handling:
# Bad: Using assert for data validation
def process_age(age):
assert age >= 0, "Age cannot be negative"
return age * 2
# Good: Using proper error handling
def process_age(age):
if age < 0:
raise ValueError("Age cannot be negative")
return age * 2
Final Takeaways
- Assert statements are debugging tools intended for developers, not end-users
- Never use assertions for data validation or business logic
- Assertions can be disabled, making them unreliable for production
- Use testing frameworks like pytest for comprehensive testing
- Keep custom error messages informative but concise
Understanding when and how to use assertions properly will improve your code quality while avoiding common pitfalls that can lead to unexpected behavior in production environments.